Create turntable cameras
This script creates a series of cameras orbiting a fixed point.
Note
This script could also be modified to create spotlights around a point.
1from p3dsdk import CameraGroup, Database, Vector3
2import ast
3import math
4
5
6def ask_vector3(prompt, default=None):
7 while True:
8 input_str = input(prompt).strip()
9 if input_str == '' and default is not None:
10 return default
11 try:
12 v = ast.literal_eval(input_str)
13 return Vector3(*v)
14 except (ValueError, TypeError):
15 print("Format should be [x, y, z] or (x, y, z)!")
16
17
18def ask_number(prompt, number_type):
19 while True:
20 input_str = input(prompt)
21 try:
22 return number_type(input_str)
23 except ValueError:
24 print("Enter a %s number!" % number_type)
25
26
27def spherical_to_cartesian(azimuth: float, elevation: float, radius: float) -> Vector3:
28 """azimuth and elevation are in degrees."""
29 azimuth_rad = math.radians(azimuth)
30 elevation_rad = math.radians(elevation)
31 x = radius * math.cos(elevation_rad) * math.cos(azimuth_rad)
32 y = radius * math.sin(elevation_rad)
33 z = radius * math.cos(elevation_rad) * math.sin(azimuth_rad)
34 return Vector3(x, y, z)
35
36
37def create_cameras(data: Database, group: CameraGroup, target: Vector3,
38 radius: float, elevation: float, steps: int):
39 assert steps > 0
40 assert radius >= 0
41 group_name = group.name if group is not None else "Turntable"
42 for i in range(steps):
43 azimuth = i * 360.0 / steps # in degrees
44 cam = data.create_camera(group)
45 cam.name = "%s_%d" % (group_name, i)
46 cam.view_from = spherical_to_cartesian(azimuth, elevation, radius)
47 cam.view_to = target
48 cam.view_up = (0, 1, 0)
49
50
51if __name__ == "__main__":
52 from p3dsdk import CurrentP3DSession
53
54 with CurrentP3DSession(fallback_port=33900) as p3d:
55 # Ask the parameters
56 target = ask_vector3("Target point (default [0, 0, 0]): ", Vector3(0, 0, 0))
57 radius = ask_number("Radius from target: ", float)
58 elevation = ask_number("Elevation (in degrees): ", float)
59 steps = ask_number("Number of cameras (steps): ", int)
60
61 # Create the camera group
62 group = p3d.data.create_camera_group("Turntable")
63
64 # Create the cameras
65 create_cameras(p3d.data, group, target, radius, elevation, steps)
Parameters
- Target
The central point around which the cameras will orbit.
- Radius
The distance between the target point and the camera. Please note that this is not the radius of the circle of cameras as it depends on elevation (except for 0° elevation).
- Elevation
Elevation of the cameras from the horizontal plane.
- Steps
The number of cameras to create. The spacing between the cameras will be
.
Spherical coordinates
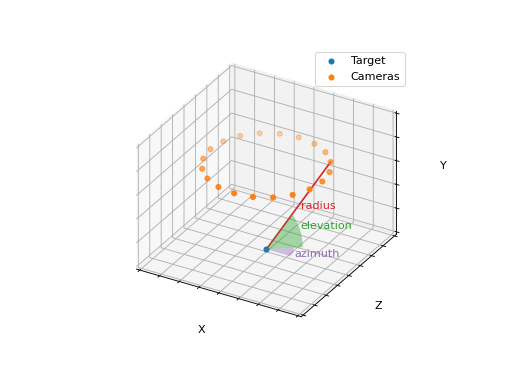
The script uses spherical coordinates to compute the camera positions. This coordinate system is defined using 3 parameters.
- Azimuth
The rotation around the vertical axis (Y axis).
in physics notation.- Elevation
The angle between the horizontal plane and the desired point.
in physics notation.- Radius
The distance of the point from the center (
).
It is possible to convert these coordinates to cartesian coordinates using these formulas.
Using spherical coordinates, it is easy to compute a circle of cameras: the azimuth is divided into steps, the elevation and radius are specified by the user.